Upload File via REST API Controller - Spring Boot Tutorial
In this video, I explain how to upload and process files using Spring Boot and REST Controller.
Topics
- Controller to process a file upload request
- Model for accepting a file and returning the response
- Configuration for media type:
multipart/form-data
Watch on YouTube:
Introduction
In modern web applications, file uploading is a common functionality that can be easily implemented using Spring Boot and REST API. In this article, we'll discuss how to create a Spring Boot application to upload files, including details about project structure, controllers, and services, and testing the functionality through Postman. We'll use an example from an actual project to understand the process.
Project Structure
In the Spring Boot project structure, all relevant parts have been updated under one package, including the newly created file package. The idea of this package is to provide features for uploading files and managing files. This includes the generic file controller, where more operations for file editing will be introduced later. The endpoint for the operation is exposed at v1 (version 1) / file one file
, and it includes one post-mapping method called upload
.
File Controller
The file controller method accepts two parameters: the file itself, of type, and a name, which is just a string. It utilizes an FileUploadRequest
object to process the file and name. The request configuration involves setting up the post-mapping to consume a multipart form of data value and produce a JSON value.
@Validated
@RestController
@RequestMapping("/v1/file")
public class FileController {
private final FileService service;
public FileController(FileService service) {
this.service = service;
}
@PostMapping(consumes = {MediaType.MULTIPART_FORM_DATA_VALUE}, produces = {MediaType.APPLICATION_JSON_VALUE})
public ResponseEntity<FileUploadResponse> upload(
@Validated @RequestParam("file") MultipartFile file,
@RequestParam("name") String name
) {
FileUploadRequest fileUploadRequest = new FileUploadRequest(file, name);
return ResponseEntity.ok(service.upload(fileUploadRequest));
}
}
File Upload Response
The file upload response includes details like ID (metadata about the file saved into the database), user-defined name, URL, and size. After parsing the file input, the data is sent to the service layer, which also has an upload operation.
Service Layer
In the service layer, a response entity is returned to indicate that the upload has succeeded. A file upload response is also returned, detailing the transformation of the uploaded file and storing some meta information. Future improvements will include saving the file in a location like GCP.
Testing Through Postman
To test the file uploading functionality, Postman is utilized. The correct headers and form data settings are applied. Postman provides a feature where the actual file can be selected and sent with the file name.
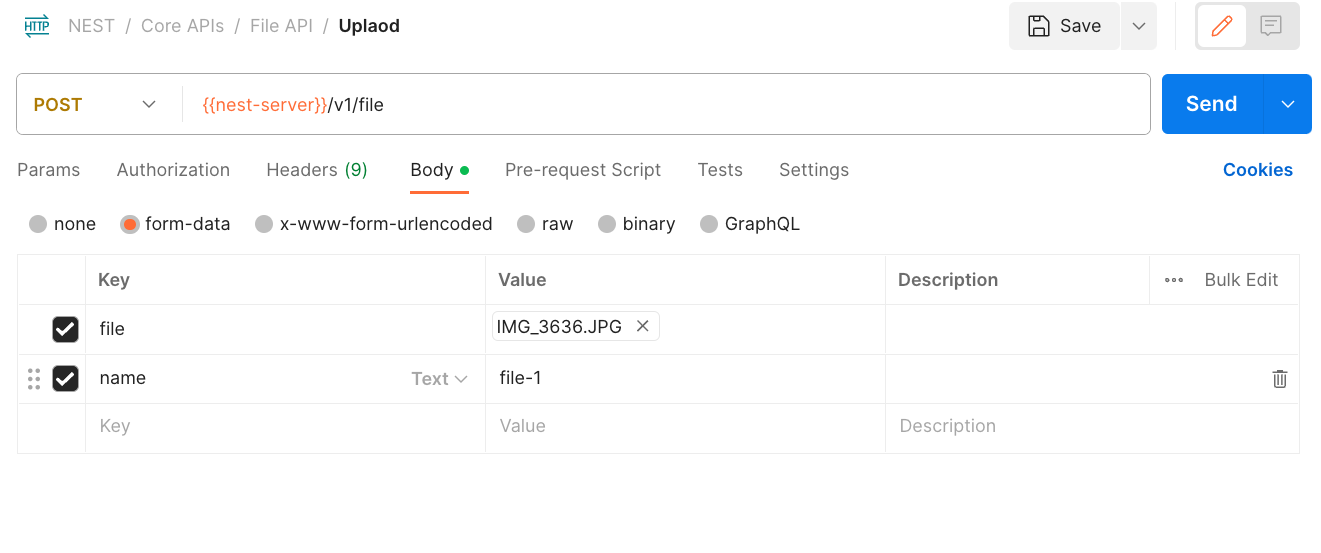
Handling the Request
During the execution, the running application and database connections are verified. The application leverages Docker Compose to manage the database, which is essential for saving metadata information about the file. The application's response includes the file ID from the database, the name sent, and the calculated size. Subsequent checks in the database confirm the record of files and metadata.
Implementation Review
To summarize, the file upload approach involves:
- Controller Setup: A controller with a post mapping that includes the consumes part (multipart form data) is essential.
- File Acceptance: Parameters to accept the file and name, along with handling the uploaded file and returning a JSON response.
- File Type: The uploaded file must be of type
MultipartFile
.
The setup is highly configurable, and additional parameters can be added to meet specific requirements.
Conclusion
Uploading files using Spring Boot and REST API is a streamlined process that leverages the powerful features of the Spring framework. By creating a well-structured project with clear controllers, services, and appropriate testing through tools like Postman, a robust file-uploading functionality can be implemented. Future enhancements can include more complex file management, editing capabilities, and integrations with different storage services. The example provided in this article should serve as a solid foundation for those looking to build or enhance file-uploading features in their applications.
* Subscribe to CoderVlogger.com and consider becoming a member.
* Check my Twitter for more up-to-date information.